One of the currently hot buzzwords is Serverless. The idea with a serverless architecture is to be able to deploy web or mobile applications without having to have a server. The name is of course a little misleading as you do need to have a server. But you do not want to manage it. And this is what is the actual goal, to deploy applications without having to manage any servers.
The first big Serverless service was AWS Simple Storage Service. S3 allowed us to server static files on the internet. We just have to upload the files and enable the proper access. We don’t have to manage servers, disks nor replication.
Serving static files is needed to deploy an application, but it is not enough. We usually also need to have a database and most certainly the ability to run code. To do this we can use the DynamoDB service for the database (There is now also a Serverless relational database option with Aurora). And Lambda for running our code.
Is Serverless application then the future? And how do we start using it? Let’s setup a simple Todo web application and have a look at how it works.
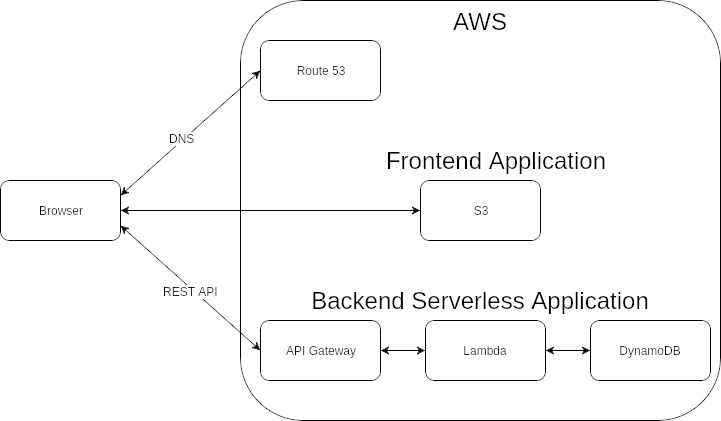
The Frontend Application
There are a lot of separate parts that are needed for a Serverless application. First we have the Frontend application. In our Todo app, we will be using a Vue application. The Frontend application works the same as any normal server based web application. The only difference here is that we use S3 to host it.
Here is the application code. It is an adaption of the todo app from http://todomvc.com/
The application is a very basic Todo app. The most interesting thing is the use for the Axios library to connect to the backend server.
addTodo() {
const value = this.newTodo && this.newTodo.trim();
const data = { text: value, completed: false };
if (!value) {
return;
}
axios.post(ENDPOINT, data)
.then((results) => {
this.todos.push(results.data);
})
.catch((e) => {
this.errors.push(e);
});
this.newTodo = '';
},
If you want to try the code on your own, remember to change the url for the backend endpoint in the constants.js
file.
Serverless Framework
The Serverless backend will need a combination of services. The API Gateway receives the request and routes it to a Lambda function. The Lambda function has the possibility to connect to the DynamoDB database.
It is possible to setup these 3 services manually. But this leads to a lot of boring repetitive work. Such as connecting API endpoints to functions and configuring IAM roles and database tables. Fortunately there is a tool that will take care of this for us. This tool is called The Serverless Framework. This framework will take care of generating a CloudFormation stack containing everything we need for our Serverless application.
Here is the code for the backend server. The backend application is based on the Pynomodb example.
The core of the app is the serverless.yml
configuration file. Here is everything needed for the backend application defined. Such as region, API endpoints and DynamoDB instance.
The configuration file connects the endpoints to functions, in our case Python functions. For example the HTTP GET
request to the path /todos
is connected to the todos/list.todo_list
function seen below.
import json
from todos.todo_model import TodoModel
import todos.config
def todo_list(event, context):
# fetch all todos from the database
results = TodoModel.scan()
# create a response
return {'statusCode': 200,
'headers': {
'Access-Control-Allow-Origin': todos.config.aws['allow-origin'],
},
'body': json.dumps({'items': [dict(result) for result in results]})}
In this function we fetch all the Todo objects from the DynamoDB and return the as a json list.
When the backend is done it can easily be deployed to AWS using the command
$ serverless deploy
Serverless: Parsing Python requirements.txt
Serverless: Installing required Python packages for runtime python3.6...
Serverless: Linking required Python packages...
Serverless: Packaging service...
Serverless: Excluding development dependencies...
Serverless: Unlinking required Python packages...
Serverless: Creating Stack...
Serverless: Checking Stack create progress...
.....
Serverless: Stack create finished...
Serverless: Uploading CloudFormation file to S3...
Serverless: Uploading artifacts...
Serverless: Uploading service .zip file to S3 (7.44 MB)...
Serverless: Validating template...
Serverless: Updating Stack...
Serverless: Checking Stack update progress...
......................................................................................................
Serverless: Stack update finished...
Service Information
service: todo-app-with-pynamodb
stage: dev
region: eu-west-1
stack: todo-app-with-pynamodb-dev
api keys:
None
endpoints:
POST - https://91jahgzne0.execute-api.eu-west-1.amazonaws.com/dev/todos
GET - https://91jahgzne0.execute-api.eu-west-1.amazonaws.com/dev/todos
GET - https://91jahgzne0.execute-api.eu-west-1.amazonaws.com/dev/todos/{todo_id}
PUT - https://91jahgzne0.execute-api.eu-west-1.amazonaws.com/dev/todos/{todo_id}
DELETE - https://91jahgzne0.execute-api.eu-west-1.amazonaws.com/dev/todos/{todo_id}
functions:
create: todo-app-with-pynamodb-dev-create
list: todo-app-with-pynamodb-dev-list
get: todo-app-with-pynamodb-dev-get
update: todo-app-with-pynamodb-dev-update
delete: todo-app-with-pynamodb-dev-delete
And the backend is deployed. Now we can take the endpoint url and configure the frontend to use it.
Here is the result http://serverlesstodo.deductivelabs.com/
Do you need help with your Serverless application? Contact us at Deductivelabs